I recently had the need to return all images that were imported into a WordPress post or page (except the featured image). Since my specific use will likely be of no use to you, I've reproduced some generic examples here of how the feature might be used.
You can find an example of how this function might be used to created an image gallery here ("Create an Image Grid Gallery With WordPress Shortcode").
Return an Array of Attached Images to a Post
The following function will return an array of all image URLs (except the featured image) attached to a post. The post ID can be the current post, or any other.
An Example Shortcode Function
The following shortcode function is pretty basic and is unlikely to serve any noble purpose. It'll simply render all the attached images (from any post) on your page.
For the purpose of providing an example, I've uploaded 5 Apollo 11 photographs. I've used the shortcode of [postimagestest]
. To return images attached to a different post, use [postimagestest id="1234"]
(where 1234 is your post ID). The result:
- https://www.beliefmedia.com.au/wp-c...nt/uploads/2017/06/apollo-11-04.jpg
- https://www.beliefmedia.com.au/wp-c...nt/uploads/2017/06/apollo-11-03.jpg
- https://www.beliefmedia.com.au/wp-c...nt/uploads/2017/06/apollo-11-02.jpg
- https://www.beliefmedia.com.au/wp-c...nt/uploads/2017/06/apollo-11-01.jpg
- https://www.beliefmedia.com.au/wp-c...nt/uploads/2017/06/apollo-11-05.jpg
For the purpose of formatting the URL (so it doesn't line wrap), I've used the beliefmedia_split_string()
function from here. I could have used WP's url_shorten()
function, but it only removes the http://www
component and truncates to 35 characters with trailing dots -- meaning all URLs would look the same. Our method preserves the filename.
Post Images (Except Features Image) in Tower
The next sample function isn't anything you would likely use. It will render all your post images (except the featured image) into your WordPress post or page with shortcode.
Copy and paste the WordPress function into your theme's functions.php
file or, if you sensibly have one installed, your custom functions plugin.
If you require shortcode to work in a sidebar widget, you'll have to enable the functionality with a filter. If you're using our custom functions plugin, you'll have that feature enabled by default.
The short snippet is the basis for creating a gallery. Of course, you would select or create an appropriate image size and then align them in paginated responsive rows. We're just outputting the thumbnail
image (automatically created when uploaded) and we're stacking them in a tower. The shortcode: [bmpostimages]
wil return the following:
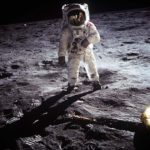
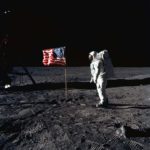
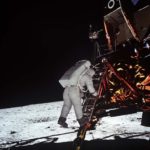
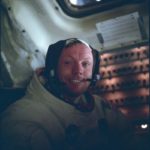
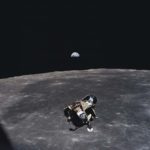
To return the images associated with another post, use [bmpostimages parent="12345"]
where 12345
is the post ID of the other post. To display a list rather than images, use [bmpostimages url="1"]
. The result of the list returns the following:
- https://www.beliefmedia.com.au/wp-c.../06/apollo-11-04.jpg
- https://www.beliefmedia.com.au/wp-c.../06/apollo-11-03.jpg
- https://www.beliefmedia.com.au/wp-c.../06/apollo-11-02.jpg
- https://www.beliefmedia.com.au/wp-c.../06/apollo-11-01.jpg
- https://www.beliefmedia.com.au/wp-c.../06/apollo-11-05.jpg
Again, it's not a real-world example, just a proof that you can use to build your own stuff.
The Image Gallery Function
We'll send people back to the following function from time-to-time. It's most often used in various image gallery features we share.
Considerations
- You can get the image title (since you have the ID) with the code of
$attachmenttitle = get_the_title($id);
. This might come in handy if you were creating an image "index". In fact, you could loop through all your published posts and generate a paginated index with nice scaled thumbnails. If the image had a title, you could display it and use it as an alt tag. - Need to search for all PDF documents attached to a page? Use
'post_mime_type' => 'application/pdf'
in your query. This should work for any file type you can upload through WP's media library. - If you wanted to print a summary of images attached to a post in a page index or similar (something I'll be doing in an upcoming project) you might consider getting image meta data with the following function (or similar):
If you wanted to test the function, use the following shortcode function so you know what kind of data the function returns:
(Close the pre tags... I've removed them for formatting).
In my case, [attachmentdatatest id="1234"]
returns the following array:
- Alternatively (to the above function, or in addition to) consider retrieving attached meta data with WP's wp_get_attachment_metadata
function (particularly if you're interested in the
image_meta
data, that, in part, returns camera type, timestamp, and shutter speed). - If uploading images that aren't attached to a parent post and you would like to emulate what we've described above, you could perhaps
explode
a string of comma separated IDs and then loop over them individually.
Download
The download includes the shortcode and other examples on this page. If you're after something specific image related, let us know.
Title: Return all Images Attached to a WordPress Post or Page
Description: Find all Images (Except the Featured Image) Attached to a WordPress Post or Page. Includes shortcode examples.
Download • Version 0.2, 1.4K, zip, Category: WordPress Shortcodes